Introduction
Nowadays the computer’s security is more and more important, you want to know who and when your files were accessed. You want to track the file I/O activities for the file audit or continue data protection application. The EaseFilter File Monitor Filter Driver SDK is a file system filter driver development kit, allow you to develop the file system monitoring software easily.
Understand The File System Filter Driver
A file system filter driver intercepts requests targeted at a file system or another file system filter driver. By intercepting the request before it reaches its intended target, the filter driver can extend or replace functionality provided by the original target of the request. File system filtering services are available through the filter manager in Windows. The Filter Manager provides a framework for developing File Systems and File System Filter Drivers without having to manage all the complexities of file I/O.
The EaseFilter File Monitor Filter Driver SDK
EaseFilter Filter Driver SDK can monitor Windows file I/O activities in real time, track the file access and changes, monitor file and folder permission changes, audit who is writing, deleting, moving or reading files, report the user name and process name, get the user name and the ip address when the Windows file server’s file is accessed by network user.
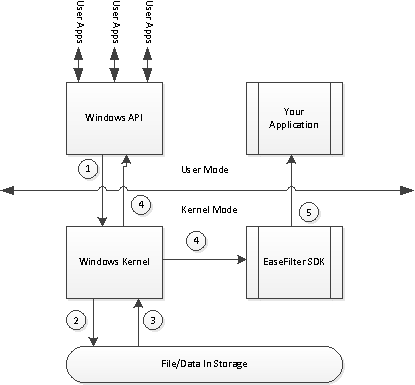
What Can You Do With File Monitor Filter Driver SDK
You can develop the software for the following purposes with the File Monitor Filter Driver SDK:
- Continuous data protection (CDP). Create your own Continuous data protection (CDP) software to log the file update information, write information with offset and length in real time.
- File Auditing. Audit your file content. You can intercept any file system call, analyze it content, log it.
- Log File Access. Create Access Log, you will know who, when, what files were accessed.
- File Journaling. Journal the file update information. This control may be based on any file parameters, such as its location, type, size, etc.
Track the file changes
With the File Monitor SDK, you can watch for changes in files and subdirectories of the specified directory. You can create a filter rule to watch files on a local computer, a network drive, or a remote computer. You can register the file change events to get the notification when the user or process changed a file.
- Monitor file creation: you will get the notification when a user/process created a new file.
- Monitor file delete: you will get the notification when a user/process deleted a file.
- Monitor file rename: you can get the notification when user/process renamed a file.
- Monitor file written: you can get the notification when a user/process wrote data to a file.
- Monitor file security changes: you can get the notification when a user/process changed a file’s security.
- Monitor file information changes: you can get the notification when a user/process changed a file’s information (file size, file time, file attributes).
Track the file I/O activities in real-time
You can register the below file I/O events to get the notification when a process requested an I/O to a file. From the return events you will know who accessed the file, the I/O request information and the I/O return status.
OnPostFileCreate: fires this event after a process opened or created a file. You will know know the create option “DesiredAccess”, “CreateDisposition”, “CreateOptions” and “ShareAccess”. You will know the create status of the operation with one of these: FILE_CREATED, FILE_OPENED, FILE_OVERWRITTEN, FILE_SUPERSEDED, FILE_EXISTS, FILE_DOES_NOT_EXIST.
OnPostFileRead: fires this event after a process read the file’s data. You will know the read offset and length, read type: cache read, non cache read or paging read.
OnPostFileWrite: fires this event after a process wrote data to a file. You will know the write offset and length, write type: cache write, non cache write or paging write.
OnPostQueryFileInfo: fires this event after a process queried file’s information. You will get the file’s allocation size, file size, file time, file attributes and file internal Id.
OnPostSetFileInfo: fires this event after a process changed a file’s information. You will know what file’s information(file size, file attributes, file time) was set to a file.
OnPostMoveOrRenameFile: fires this event after a process renamed a file. You will know the old file name and the new file name.
OnPostDeleteFile: fires this event after a process deleted a file. You will know the deleted file name.
OnPostQueryDirectoryFile: fires this event after a process browsed a directory. You will get the file list of the directory.
OnPostQueryFileSecurity: fires this event after a process queried a file’s security information. You will get the file’s security information.
OnPostSetFileSecurity: fires this event after a process changed a file’s security information. You will know the file’s security information set to the file.
OnPostFileHandleClose: fires this event after a process closed a file’s handle.
How To Use The EaseFilter File Monitor Filter Driver SDK
It is simple to use the EaseFilter File Monitor Filter Driver SDK. The following example creates a filter rule to watch the directory specified at run time. The component is set to watch for all file change in the directory. If a file was changed, the file name, file change type, user name, process name will be printed to the console. The component also is set to watch the file open and file read IO, the IO was triggered, the file open and file read information will be printed to the console.
using System;
using EaseFilter.FilterControl;
namespace FileMonitorConsole
{
class Program
{
static FilterControl filterControl = new FilterControl();
static void Main(string[] args)
{
string lastError = string.Empty;
string licenseKey = "**************************";
FilterAPI.FilterType filterType = FilterAPI.FilterType.MONITOR_FILTER;
int serviceThreads = 5;
int connectionTimeOut = 10; //seconds
try
{
if (!filterControl.StartFilter(filterType, serviceThreads, connectionTimeOut, licenseKey, ref lastError))
{
Console.WriteLine("Start Filter Service failed with error:" + lastError);
return;
}
//the watch path can use wildcard to be the file path filter mask.i.e. '*.txt' only monitor text file.
string watchPath = "c:\\test\\*";
if (args.Length > 0)
{
watchPath = args[0];
}
//create a file monitor filter rule, every filter rule must have the unique watch path.
FileFilter fileMonitorFilter = new FileFilter(watchPath);
//Filter the file change event to monitor all file change events.
fileMonitorFilter.FileChangeEventFilter = FilterAPI.MonitorFileEvents.NotifyAll;
//register the file change callback events.
fileMonitorFilter.NotifyFileWasChanged += NotifyFileChanged;
//Filter the monitor file IO events
fileMonitorFilter.MonitorFileIOEventFilter = (ulong)(MonitorFileIOEvents.OnFileOpen | MonitorFileIOEvents.OnFileRead);
fileMonitorFilter.OnFileOpen += OnFileOpen;
fileMonitorFilter.OnFileRead += OnFileRead;
filterControl.AddFilter(fileMonitorFilter);
if (!filterControl.SendConfigSettingsToFilter(ref lastError))
{
Console.WriteLine("SendConfigSettingsToFilter failed." + lastError);
return;
}
Console.WriteLine("Start filter service succeeded.");
// Wait for the user to quit the program.
Console.WriteLine("Press 'q' to quit the sample.");
while (Console.Read() != 'q') ;
filterControl.StopFilter();
}
catch (Exception ex)
{
Console.WriteLine("Start filter service failed with error:" + ex.Message);
}
}
/// Fires this event when the file was changed.
static void NotifyFileChanged(object sender, FileChangeEventArgs e)
{
Console.WriteLine("NotifyFileChanged:" + e.FileName + ",eventType:" + e.eventType.ToString()
+ ",userName:" + e.UserName + ",processName:" + e.ProcessName);
}
/// Fires this event after the file was opened, the handle is not closed.
static void OnFileOpen(object sender, FileCreateEventArgs e)
{
Console.WriteLine("FileOpen:" + e.FileName + ",status:" + e.IOStatusToString()
+ ",userName:" + e.UserName + ",processName:" + e.ProcessName);
}
/// Fires this event after the read IO was returned.
static void OnFileRead(object sender, FileReadEventArgs e)
{
Console.WriteLine("FileRead:" + e.FileName + ",offset:" + e.offset + ",readLength:"
+ e.returnReadLength + ",userName:" + e.UserName + ",processName:" + e.ProcessName);
}
}
}