Introduction
Transparent File Encryption is a method of encrypting file at rest, where the encryption and decryption process is transparent to the users and the applications. It means that the user or application does not need to take any explicit action to encrypt or decrypt the data. This allows you to implement the encryption solution without changing your existing systems.
Understand The File System Filter Driver
A file system filter driver intercepts requests targeted at a file system or another file system filter driver. By intercepting the request before it reaches its intended target, the filter driver can extend or replace functionality provided by the original target of the request. File system filtering services are available through the filter manager in Windows. The Filter Manager provides a framework for developing File Systems and File System Filter Drivers without having to manage all the complexities of file I/O.

Transparent On-Access File Level Encryption
The EaseFilter Encryption Filter Driver(EEFD) is a transparent on-access file level encryption file system filter driver. It can encrypt or decrypt the file automatically in the file system level. The EEFD is a mature commercial product. It provides a complete modular framework for the developers to build the on access file encryption software. With the EEFD, you can incorporate transparent on-access, file level encryption into your application.
The EEFD can encrypt the new created file automatically. Firstly, the EEFD will encrypt the data before the applications write the data to the disk. After that when the applications read the encrypted file, the EEFD will decrypt the data automatically. The encryption and decryption process are transparent to the application. The encryption and decryption process won’t generate the temporary files. The encrypted file always stay encrypted in the disk. Only the authorized users or processes can see the clear data of the encrypted file.
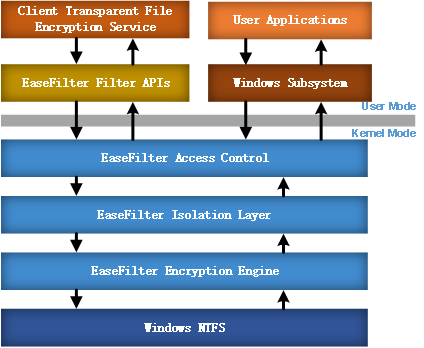
AES Encryption Algorithm
The EaseFilter Encryption Filter Driver encrypts the files with the AES algorithm, implementing with a build in Microsoft CNG encryption library. The AES Encryption algorithm (also known as the Rijndael algorithm) is a specification for the encryption of electronic data established by the U.S. National Institute of Standards and Technology (NIST) in 2001. AES is a US FIPS 140-2 compliant symmetric block cipher algorithm. It has a fixed block size of 128 bits, and different key size of 128, 192, or 256 bits.
Authorize The Encrypted File Access To The Specific Processes or Users
In Windows, when an application opens a file with memory mapping, like notepad, the file system will map the file data into the memory and will share the data with other applications. For encrypted file, when an authorized process opened an encrypted file, you don’t want an unauthorized process to read this file even though this file’s data already in memory. The EEFD supports the per process access restriction for the on access file encryption. In EEFD every encrypted file open, the system will create a unique private memory section, it won’t be shared with other applications.
The EEFD utilizes the Isolation Mini Filter Driver technology to implement two views of the encrypted file to the process. The unauthorized process will see the encrypted data view with the raw ciphertext. The authorized processes will see the decrypted data view with the plaintext.
You an setup the whitelist or blacklist of the processes to the encrypted files. The whitelist process can read the encrypted file to get the clear text. The blacklist process only can get the encrypted raw data. You can authorize the encrypted file access to the specific processes or users.

Secure File Sharing with DRM Protection
The EaseFilter Encryption Filter Driver can encrypt the file with the appended header. With the encrypted file header, you can embed the DRM data to the header. So the encrypted file supports the DRM data embedding, you can share the encrypted file securely, and the file stays encrypted in transit. You can implement the secure file sharing solution easily. You can grant, revoke or expire the access to the shared encrypted file at any time, even after the encrypted file has been sent out of your organization.

A C# File Encryption Example
The EaseFilter Encryption Filter Driver SDK provides C++ and C# demo source code, Implementing file encryption application in C# with EEFD SDK is simple. The below C# code snippet will setup two filter rules, one filter rule for encryption, only the authorized processes can read the encrypted files. The second filter rule for decryption, only the authorized users or processes can decrypt the files.
using System;
using EaseFilter.FilterControl;
namespace AutoFileEncryption
{
class Program
{
static FilterControl filterControl = new FilterControl();
static void Main(string[] args)
{
string lastError = string.Empty;
string licenseKey = "Email us to request a trial key: info@easefilter.com";
FilterAPI.FilterType filterType = FilterAPI.FilterType.CONTROL_FILTER
| FilterAPI.FilterType.ENCRYPTION_FILTER | FilterAPI.FilterType.PROCESS_FILTER;
int serviceThreads = 5;
int connectionTimeOut = 10; //seconds
try
{
//copy the right Dlls to the current folder.
Utils.CopyOSPlatformDependentFiles(ref lastError);
if (!filterControl.StartFilter(filterType, serviceThreads, connectionTimeOut, licenseKey, ref lastError))
{
Console.WriteLine("Start Filter Service failed with error:" + lastError);
return;
}
//setup a file filter rule for folder encryptFolder
string encryptFolder = "c:\\encryptFolder\\*";
FileFilter fileFilter = new FileFilter(encryptFolder);
//enable the encryption for the filter rule.
fileFilter.EnableEncryption = true;
//get the 256bits encryption key with the passphrase
string passPhrase = "mypassword";
fileFilter.EncryptionKey = Utils.GetKeyByPassPhrase(passPhrase, 32);
//disable the decyrption right, read the raw encrypted data for all except the authorized processes or users.
fileFilter.EnableReadEncryptedData = false;
//setup the authorized processes to decrypt the encrypted files.
string authorizedProcessesForEncryptFolder = "notepad.exe;wordpad.exe";
string[] processNames = authorizedProcessesForEncryptFolder.Split(new char[] { ';' });
if (processNames.Length > 0)
{
foreach (string processName in processNames)
{
if (processName.Trim().Length > 0)
{
//authorized the process with the read encrypted data right.
fileFilter.ProcessNameAccessRightList.Add(processName, FilterAPI.ALLOW_MAX_RIGHT_ACCESS);
}
}
}
//setup the authorized users to decrypt the encrypted files.
string authorizedUsersForEncryptFolder = "domainName\\user1";
if (!string.IsNullOrEmpty(authorizedUsersForEncryptFolder) && !authorizedUsersForEncryptFolder.Equals("*"))
{
string[] userNames = authorizedUsersForEncryptFolder.Split(new char[] { ';' });
if (userNames.Length > 0)
{
foreach (string userName in userNames)
{
if (userName.Trim().Length > 0)
{
//authorized the user with the read encrypted data right.
fileFilter.userAccessRightList.Add(userName, FilterAPI.ALLOW_MAX_RIGHT_ACCESS);
}
}
}
if (fileFilter.userAccessRightList.Count > 0)
{
//set black list for all other users except the white list users.
uint accessFlag = FilterAPI.ALLOW_MAX_RIGHT_ACCESS & ~(uint)FilterAPI.AccessFlag.ALLOW_READ_ENCRYPTED_FILES;
//disable the decryption right, read the raw encrypted data for all except the authorized users.
fileFilter.userAccessRightList.Add("*", accessFlag);
}
}
//add the encryption file filter rule to the filter control
filterControl.AddFilter(fileFilter);
//setup a file filter rule for folder decryptFolder
string decryptFolder = "c:\\decryptFolder\\*";
FileFilter decryptFileFilter = new FileFilter(decryptFolder);
//enable the encryption for the filter rule.
decryptFileFilter.EnableEncryption = true;
//get the 256bits encryption key with the passphrase
decryptFileFilter.EncryptionKey = Utils.GetKeyByPassPhrase(passPhrase, 32);
//don't encrypt the new created file in the folder.
decryptFileFilter.EnableEncryptNewFile = false;
//disable the decyrption right, read the raw encrypted data for all except the authorized processes or users.
decryptFileFilter.EnableReadEncryptedData = false;
//setup authorized processes to decrypt the encrypted files.
string authorizedProcessesForDecryptFolder = "notepad.exe;wordpad.exe";
processNames = authorizedProcessesForDecryptFolder.Split(new char[] { ';' });
if (processNames.Length > 0)
{
foreach (string processName in processNames)
{
if (processName.Trim().Length > 0)
{
//authorized the process with the read encrypted data right.
decryptFileFilter.ProcessNameAccessRightList.Add(processName, FilterAPI.ALLOW_MAX_RIGHT_ACCESS);
}
}
}
filterControl.AddFilter(decryptFileFilter);
if (!filterControl.SendConfigSettingsToFilter(ref lastError))
{
Console.WriteLine("SendConfigSettingsToFilter failed." + lastError);
return;
}
Console.WriteLine("Start filter service succeeded.");
// Wait for the user to quit the program.
Console.WriteLine("Press 'q' to quit the sample.");
while (Console.Read() != 'q') ;
filterControl.StopFilter();
}
catch (Exception ex)
{
Console.WriteLine("Start filter service failed with error:" + ex.Message);
}
}
}
}